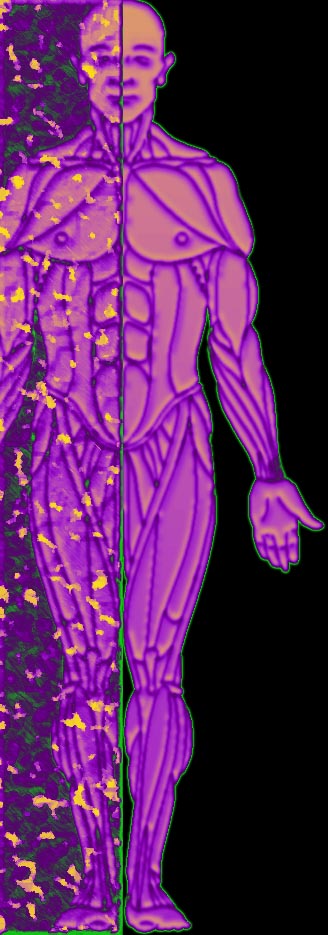 |
|
|
'Mouse Rays in 3D
'J. Eric Coleman
'
Public Sub MouseGetRay()
'#####'
'Purpose: This sub will take screen coordinates of your mouse and extend a ray
' untill it hits the X,Y plane, (where Z = 0). You can modify this
' to check for collision detection with objects in your scene. Just follow
' the ray from the begining to the end and do your collision detection.
'
' A word of caution: This function uses the near and far clippling planes
' that you define in your projection matrix. Do NOT define a near clipping
' plane of 0. That will cause all kinds of nasty divide by zero errors all
' throughout directx, not just here. Setting the near clipping plane to 1 is good.
'#####'
Dim dx As Single, dy As Single
Dim pi3 As Single
pi3 = 3.1415926 / 3 'this is the aspect ratio that I use,
'replace this with what you use.
Dim width As Single, height As Single
width = g_mode.lWidth 'get the current width and height of our screen mode.
height = g_mode.lHeight
'Fancy math that you probably wouldn't understand anyway
dx = Tan(pi3 * 0.5) * (g_x / (width * 0.5) - 1#) * 1.333333 '1.333 is the aspect ratio
dy = Tan(pi3 * 0.5) * (1# - g_y / (height * 0.5))
Dim p1 As d3dvector4
Dim p2 As d3dvector4
Dim p3 As D3DVECTOR
p1.X = dx * g_Near 'g_near is the Near clipping plane
p1.Y = dy * g_Near
p1.Z = g_Near
p1.w = 1
p2.X = dx * g_Far 'g_far is the Far clipping plane
p2.Y = dy * g_Far
p2.Z = g_Far
p2.w = 1
Dim Iv As D3DMATRIX 'this variable will be the inverted matrix
InverseMatrix Iv, g_ViewMatrix
p1 = vM(p1, Iv) 'vM is vector times matrix, see other article for this code.
p2 = vM(p2, Iv)
'That's it. Everything that follows is code that I use to intersect the (x,y) plane.
'With the two points p1 and p2, you have your ray extending out from your mouse passing
'through the near and far clipping planes that you set up.
'## Optional ##'
p3.X = p2.X - p1.X
p3.Y = p2.Y - p1.Y
p3.Z = p2.Z - p1.Z
If p3.Z = 0 Then
g_vectorCursor.X = g_vectUp.X
g_vectorCursor.Y = g_vectUp.Y
g_vectorCursor.Z = g_vectUp.Z
Else
g_vectorCursor.X = p1.X + (-p1.Z / p3.Z) * p3.X
g_vectorCursor.Y = p1.Y + (-p1.Z / p3.Z) * p3.Y
g_vectorCursor.Z = p1.Z + (-p1.Z / p3.Z) * p3.Z
End If
'## End of Optional ##'
End Sub
|
|